What is an Array of Objects?
Unlike traditional array which store values like string, integer, Boolean, etc. array of objects stores objects. The array elements store the location of reference variables of the object
Syntax:
Class obj[]= new Class[array_length]
Example: To create Array Of Objects
Step 1) Copy the following code into an editor
Step 1) Copy the following code into an editor
class ObjectArray{ public static void main(String args[]){ Account obj[] = new Account[2] ; //obj[0] = new Account(); //obj[1] = new Account(); obj[0].setData(1,2); obj[1].setData(3,4); System.out.println("For Array Element 0"); obj[0].showData(); System.out.println("For Array Element 1"); obj[1].showData(); } } class Account{ int a; int b; public void setData(int c,int d){ a=c; b=d; } public void showData(){ System.out.println("Value of a ="+a); System.out.println("Value of b ="+b); } }
Step 2) Save , Compile & Run the Code.
Step 3) Error=? Try and debug before proceeding to step 4.
Step 4) The line of code, Account obj[] = new Account[2]; exactly creates an array of two reference variables as shown below
Step 3) Error=? Try and debug before proceeding to step 4.
Step 4) The line of code, Account obj[] = new Account[2]; exactly creates an array of two reference variables as shown below
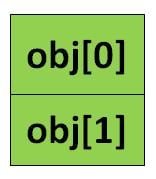
Step 5) Uncomment Line # 4 & 5. This step creates objects and assigns them to the reference variable array as shown below. Your code must run now.
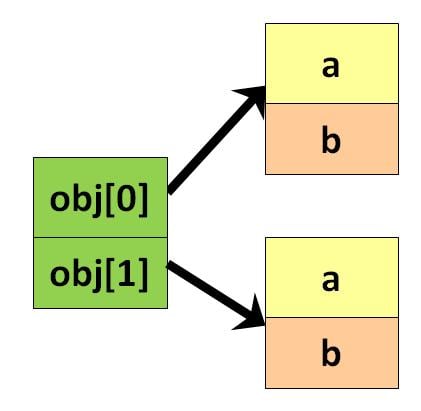
Output:
For Array Element 0 Value of a =1 Value of b =2 For Array Element 1 Value of a =3 Value of b =4
No comments:
Post a Comment