What is Hashmap in Java?
A HashMap basically designates unique keys to corresponding values that can be retrieved at any given point.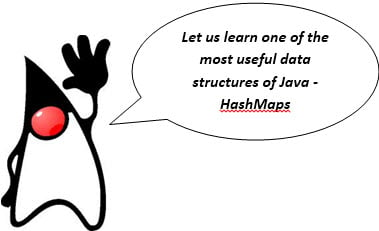
Features of Java Hashmap
a) The values can be stored in a map by forming a key-value pair. The value can be retrieved using the key by passing it to the correct method.b) If no element exists in the Map, it will throw a ‘NoSuchElementException’.
c) HashMap stores only object references. That is why, it is impossible to use primitive data typeslike double or int. Use wrapper class (like Integer or Double) instead.
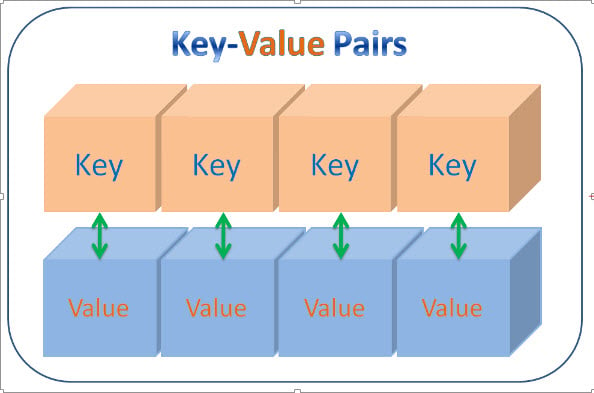
Using HashMaps in Java Programs:
Following are the two ways to declare a Hash Map:HashMap<String, Object> map = new HashMap<String, Object>(); HashMap x = new HashMap();Important Hashmap Methods
- get(Object KEY) – This will return the value associated with a specified key in this Java hashmap.
- put(Object KEY, String VALUE) – This method stores the specified value and associates it with the specified key in this map.
Java Hashmap Example
Following is a sample implementation of java Hash Map:import java.util.HashMap; import java.util.Map; public class Sample_TestMaps{ public static void main(String[] args){ Map<String, String> objMap = new HashMap<String, String>(); objMap.put("Name", "Suzuki"); objMap.put("Power", "220"); objMap.put("Type", "2-wheeler"); objMap.put("Price", "85000"); System.out.println("Elements of the Map:"); System.out.println(objMap); } }
Output:
Elements of the Map: {Type=2-wheeler, Price=85000, Power=220, Name=Suzuki}
Example 2: Remove a value from HashMap based on key
import java.util.*; public class HashMapExample { public static void main(String args[]) { // create and populate hash map HashMap<Integer, String> map = new HashMap<Integer, String>(); map.put(1,"Java"); map.put(2, "Python"); map.put(3, "PHP"); map.put(4, "SQL"); map.put(5, "C++"); System.out.println("Tutorial in Guru99: "+ map); // Remove value of key 5 map.remove(5); System.out.println("Tutorial in Guru99 After Remove: "+ map); } }
Output:
Tutorial in Guru99: {1=Java, 2=Python, 3=PHP, 4=SQL, 5=C++} Tutorial in Guru99 After Remove: {1=Java, 2=Python, 3=PHP, 4=SQL}
Lets us ask a few queries to the Hash Map itself to know it better
Q: So Mr.Hash Map, how can I find if a particular key has been assigned to you?
A: Cool, you can use the containsKey(Object KEY) method with me, it will return a Boolean value if I have a value for the given key.
Q: How do I find all the available keys that are present on the Map?
A: I have a method called as keyset() that will return all the keys on the map. In the above example, if you write a line as – System.out.println(objMap.keySet());
It will return an output as-
[Name, Type, Power, Price]
Similarly, if you need all the values only, I have a method of values().
System.out.println(objMap.values());
It will return an output as-
[Suzuki, 2-wheeler, 220, 85000]
Q: Suppose, I need to remove only a particular key from the Map, do I need to delete the entire Map?
A: No buddy!! I have a method of remove(Object KEY) that will remove only that particular key-value pair.
Q: How can we check if you actually contain some key-value pairs?
A: Just check if I am empty or not!! In short, use isEmpty() method against me ;)
No comments:
Post a Comment